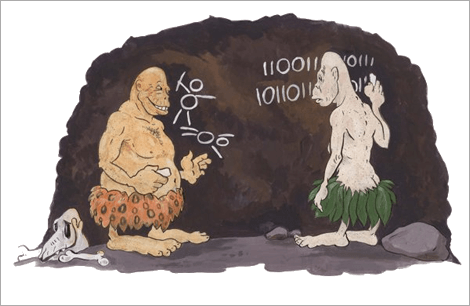
Every so often it’s good practice to review the methods available in your framework just in case there is an overlooked method already available for something you are trying to do — even if it is code you’ve repeated numerous times. Actually especially when it is code you have repeated numerous times.
For me, evaluating C# strings had become such a repeatable process that for the longest time I had overlooked the two simple methods below:
Method #1 Utilize string.IsNullOrEmpty() Whenever Possible
Normally I would use the following if statement when I wanted to check if a string was returning a null or empty value:
{
do something blah, blah
}
That same task however can be accomplished using the string.IsNullOrEmpty() method:
{
do something better blah, blah, blah
}
If you want to take that a step further, the string.IsNullOrWhiteSpace() method will not only check if your string is empty or null, but it will also make sure it does not contain any whitespace characters so you don’t have to use any trim functions.
Here is an example:
{
do something even better blah, blah, blah
}
Keep in mind though, the string.IsNullOrWhiteSpace() method is only provided in version 4.0 and higher of the .NET framework.
Method #2 When Comparing Two Strings use String.Compare instead of ==
Speaking of null string references, another thing to be mindful of is using the equality symbol == when comparing strings. A better approach is to use the String.Compare method because it can handle null string references and will also ignore case when comparing strings.
So instead of…
Try using…
Bottom line… occasionally try spending a few extra minutes and explore the different methods that will come up as options in Visual Studio Intellisense. When you see one you are unfamiliar with, Google it and see if it can save you time in future applications.